软件设计模式中的最简单的设计模式的实现,主要是应用在线程池中。分为单例模式介绍、线程池实现两部分
1 单例模式
单例模式(Singleton Pattern)是软件设计模式中最简单的设计模式之一。这种类型的设计模式属于创建型模式,它提供了一种创建对象的最佳方式。
这种模式涉及到一个单一的类,该类负责创建自己的对象,同时确保只有单个对象被创建。这个类提供了一种访问其唯一的对象的方式,可以直接访问,不需要实例化该类的对象。
单例模式是一种创建型设计模式,它确保一个类只有一个实例,并提供了一个全局访问点来访问该实例。
注意:
1、单例类只能有一个实例。
2、单例类必须自己创建自己的唯一实例。
3、单例类必须给所有其他对象提供这一实例。
单例模式图如下:
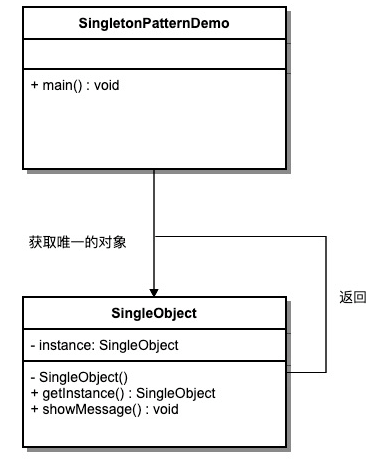
简要的示例如下:
- 懒汉版:单例实例在第一次被使用时才进行初始化,这叫做延迟初始化。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Singleton { private: static Singleton* instance; private: Singleton() {}; ~Singleton() {}; Singleton(const Singleton&); Singleton& operator=(const Singleton&); public: static Singleton* getInstance() { if(instance == NULL) instance = new Singleton(); return instance; } };
Singleton* Singleton::instance = NULL;
|
但是这样做有内存泄漏的问题,有两种解决办法:
- 智能指针
- 静态的嵌套类的对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| class Singleton { private: static Singleton* instance; private: Singleton() { }; ~Singleton() { }; Singleton(const Singleton&); Singleton& operator=(const Singleton&); private: class Deletor { public: ~Deletor() { if(Singleton::instance != NULL) delete Singleton::instance; } }; static Deletor deletor; public: static Singleton* getInstance() { if(instance == NULL) { instance = new Singleton(); } return instance; } };
Singleton* Singleton::instance = NULL; Singleton::Deletor Singleton::deletor;
|
- 饿汉版
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class Singleton { private: static Singleton instance; private: Singleton(); ~Singleton(); Singleton(const Singleton&); Singleton& operator=(const Singleton&); public: static Singleton& getInstance() { return instance; } }
Singleton Singleton::instance;
|
2 在线程池中实现单例模式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| template <typename T> class threadpool { private: static threadpool* instance; public: static threadpool* getInstance() { if(instance == NULL) instance = new threadpool(); return instance; } bool append(T *request, int state); bool append_p(T *request); private: threadpool(int actor_model, connection_pool *connPool, int thread_number = 8, int max_request = 10000); ~threadpool(); private: static void *worker(void *arg); void run();
private: int m_thread_number; int m_max_requests; pthread_t *m_threads; std::list<T *> m_workqueue; locker m_queuelocker; sem m_queuestat; connection_pool *m_connPool; int m_actor_model; };
|
感谢看到这里,在记录中收获成长,道阻且长